The main idea of the abstract class lies in the following thesis: sometimes not ready-made classes are required, but in a “raw” form. Such blanks cannot be directly used (instantiated).

What is the abstract class in Java?
Consider another example.There is an abstract Calendar class in Java in the Java.util package. It does not implement a specific calendar, which is used, for example, in Western and Eastern Europe, China, North Korea, Thailand, etc. But it has many useful functions, for example, adding a few days to a specific date: these functions are required for any calendar implementation. You cannot generate an instance from an abstract class.
Abstract classes, abstract Java methods
Допустим, нужно разработать несколько графических elements, for example, geometric shapes: a circle, a rectangle, a star, etc. And there is a container that draws them. Each component has a different appearance, so the corresponding method (let it be called paint) is implemented differently. However, each component has many common features: the figures must be inscribed in a rectangle, they may have color, be visible and invisible, etc. That is, you need to create a parent class for all these figures, where each component will inherit common properties.

If a class has abstract methods, thenclass is abstract. The word class is preceded by the keyword abstract, and in the method header too. After the header of this method you need to put a semicolon. In Java, an abstract class cannot spawn instances. If we want to prohibit their creation, even if the class does not have abstract methods, then the class can be declared abstract. But if a class has at least one abstract method, then the class must be abstract. It is impossible that the class was both abstract and final, and the method too. The method cannot be abstract, private, static, native. In order for the heir classes to be declared non-abstract and instantiate them, they must implement all the abstract methods of the parent. The class itself can use its abstract methods.
Example:
- abstract class AClass {
- public abstract void method (int a);
- }
- class BClass extends AClass {
- public void method (int a) {
- // body
- }
Variables of the abstract class type are allowed. They can refer to a non-abstract descendant of this class or be null.
Interfaces in Java - an alternative to multiple inheritance
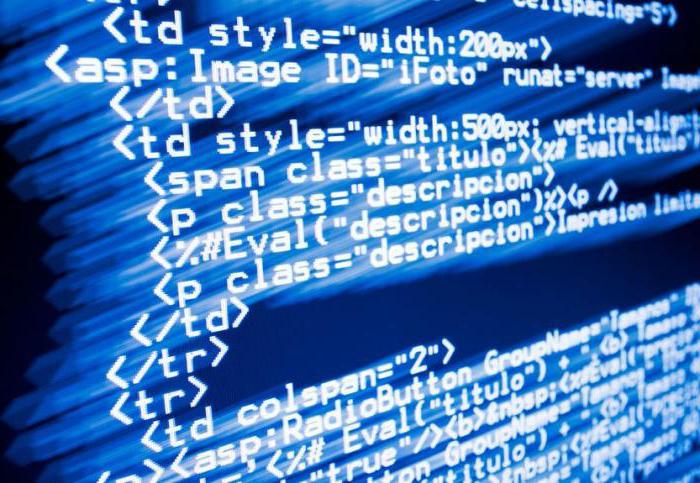
In Java, there is no multiple inheritance, because then certain problems arise. A class cannot inherit from multiple classes. But then he can implement several interfaces.
Interfaces and Java Abstract Classes - Conceptssimilar but not identical. The interface can be declared as public, then it is accessible to all, or the public modifier can be omitted, then the interface is available only within its package. The abstract keyword is not required, since the interface is already abstract, but it can be specified.
Interface declaration

It starts with a headline and can go firstthe keyword public, then the word interface. Then there can be the word extends and the enumeration of the interfaces from which the given is inherited. Here repetitions are not allowed, and it is also impossible that the inheritance relationship forms a cyclical dependency. Then comes the interface body, enclosed in braces. The following elements are declared in the interface body: constant fields and abstract methods. All fields are public final static - all these modifiers are optional. All methods are considered public abstract - these modifiers can also be specified. Now quite enough is said about the difference between the abstract class and the Java interface.
- public interface AI extends B, C, D {
- // body
- }
To declare a class as an interface inheritor, you must use the implements keyword:
- class AClass implements BI, CI, DI {}
That is, if an interface name is specified in the class declaration after implements, the class implements it. The heirs of this class get its elements, so they also implement it.
Interface type variables are also allowed.They can refer to the type of the class that implements this interface, or null. Such variables have all the elements of the class Object, because objects are generated from classes, and those, in turn, are inherited from the class Object.
In this article, we looked at some of the elements of the Java object model — abstract classes, abstract methods, and interfaces.